Convex hull Algorithm
2020-1ํ๊ธฐ, ๋ํ์์ โ์๊ณ ๋ฆฌ์ฆโ ์์ ์ ๋ฃ๊ณ ๊ณต๋ถํ ๋ฐ๋ฅผ ์ ๋ฆฌํ ๊ธ์ ๋๋ค. ์ง์ ์ ์ธ์ ๋ ํ์์ ๋๋ค :)
Definition. Convex hull
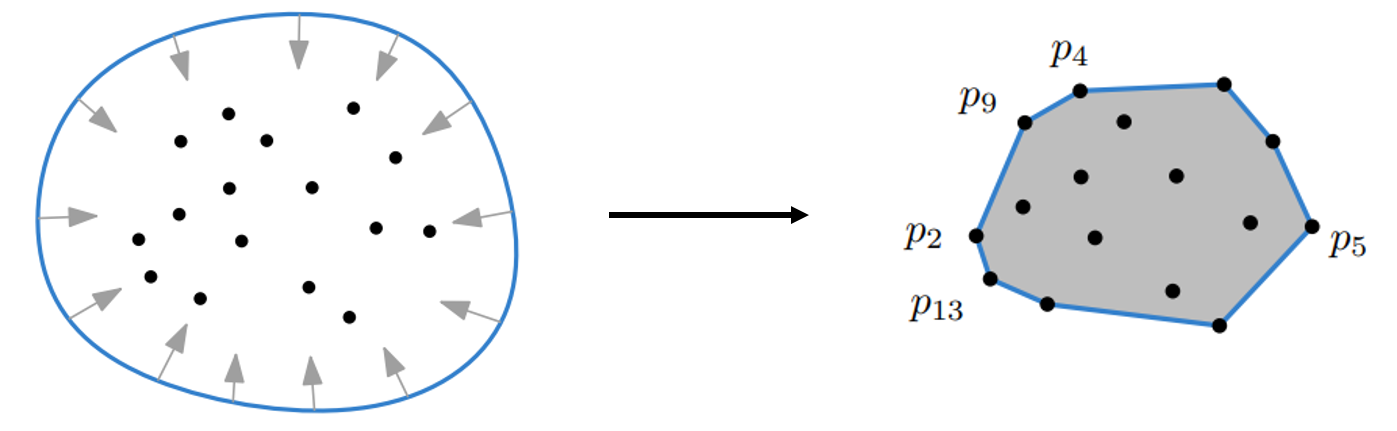
The <convex hull> of a set $P$ of points in the plane is the smallest convex set containing $P$.
Equivalently, it is the largest convex polygon whose vertices are points in $P$.
-
inputs: a set $P = \{ p_1, p_2, \dots, p_n \}$ of points, where $p_i = (x_i, y_i)$
-
output: $(p_2, p_9, \dots, p_5, \dots, p_13)$, a representation of the convex hull
๐ฅ NOTE: We assume that the points in $P$ are in general position, meaning that no three points lie on a common line.
<Convex hull Algorithm>์ ์ดํด๋ณด๊ธฐ ์ ์ ๋จผ์ ์๋์ ๋ฌธ์ ๋ฅผ ํ์ด๋ณด์.
For three points $p$, $q$, $r$, how do we test whether $r$ lies to the left or to the right of the directed line $\vec{pq}$?
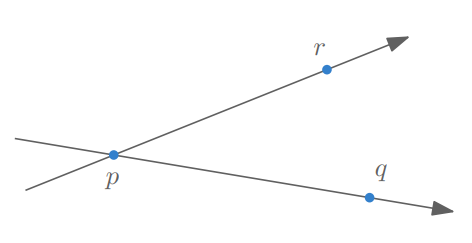
ํด๊ฒฐ ๋ฐฉ๋ฒ์ ์์ธ๋ก ๊ฐ๋จํ๋ค!! ๊ทธ๋ฅ $\vec{pq}$๋ฅผ ์ง๋๋ ์ง์ ๊ณผ $\vec{pr}$์ ์ง๋๋ ์ง์ ์ ๊ธฐ์ธ๊ธฐ๋ฅผ ๋น๊ตํด๋ณด๋ฉด ๋๋ค!!
\[\frac{q_y - p_y}{q_x - p_x} \quad \text{vs.} \quad \frac{r_y - p_y}{r_x - p_x}\]์ด๋, ์์ ๋๋์ ์ด ์๋ ๊ฒ์ โdivide by zeroโ์ ์ํ์ด ์๊ธฐ ๋๋ฌธ์, ์์ ๊ณฑ์ ์ ํํ๋ก ๋ฐ๊ฟ์ค๋ค.
\[(r_x - p_x) \cdot (q_y - p_y) \quad \text{vs.} \quad (q_x - p_x) \cdot (r_y - p_y)\]๋ง์ฝ, ์ $r$์ด ์ง์ $\vec{pq}$์ ์ผ์ชฝ์ ์๋ค๋ฉด, ์์ ๊ทธ๋ฆผ์์๋ ๋ณผ ์ ์๋ฏ์ด, $\vec{pr}$์ ๊ธฐ์ธ๊ธฐ๊ฐ $\vec{pq}$ ๋ณด๋ค ํฌ๊ฒ ๋๋ค. ๋ฐ๋ผ์,
\[\frac{q_y - p_y}{q_x - p_x} \quad < \quad \frac{r_y - p_y}{r_x - p_x}\] \[(r_x - p_x) \cdot (q_y - p_y) \quad < \quad (q_x - p_x) \cdot (r_y - p_y)\]Brute Force
์ด๋ค ๋ ์ $p$, $q$๊ฐ convex hull์ ์ด๋ฃฌ๋ค๊ณ ์๊ฐํด๋ณด์, ๊ทธ๋ฌ๋ฉด $\vec{pq}$์ ๋ํด ๋ค๋ฅธ ๋ชจ๋ ์ ๋ค์ $\vec{pq}$์ ์ค๋ฅธํธ์ ์กด์ฌํ๊ฒ ๋๋ค.
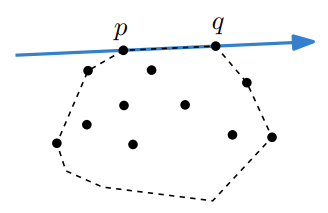
๋ง์ฝ ์ด ๊ณผ์ ์ naive ํ๊ฒ ์งํํ๋ค๋ฉด,
-
Pick two points from points set: $\displaystyle\binom{n}{2}$
-
Scan other points to determine they are in the right-side: $n$
๊ทธ๋์ ์๊ฐ ๋ณต์ก๋๋ $O(n^3)$์ด ๋๋ค.
Grahamโs Scan
$O(n^3)$ ์์ค์ ์๊ณ ๋ฆฌ์ฆ์ ์์ง ๋ง์ด ๋๋ฆฌ๋ค. <Grahamโs Scan>์ points๋ฅผ ์ ๋ ฌํ๊ณ , ๋ ๋ช๊ฐ์ง ๊ท์น์ ํตํด $O(n^3)$๋ณด๋ค ํจ์ฌ ๋น ๋ฅธ ์๊ณ ๋ฆฌ์ฆ์ ์ ์ํ๋ค.
Algorithm.
// initialization
Sort points in $P$ by x-coord and y-coord: $O(n \log n)$
Let $p, q, r$ be the leftmost three points in the sorted list $L$.
Repeat this:
โโ If $r$ lies to the right of $\vec{pq}$:
โโโโ we move one step forward in $L$.
โโ Otherwise:
โโโโ remove $q$ from the sorted list and move one step backward in $L$ if possible.
๊ธ๋ก ์ดํด๋ณด๋ฉด ์๊ณ ๋ฆฌ์ฆ์ ํ๋์ด ์ ๊ทธ๋ ค์ง์ง ์๋๋ค. ์ผ๋จ ์ฉ์ด๋ฅผ ์กฐ๊ธ ๋ค๋ฌ์ผ๋ฉด,
- $r$๋ ์ฐ๋ฆฌ๊ฐ ์ดํด๋ณด๋ ์ง์ $\vec{pq}$์ ๋ฐ๋ก ๋ค์ ์ ๋ ฌ๋ point
-
$q$๋ ์ฐ๋ฆฌ์ ์ง์ $\vec{pq}$์ ๋์ ์ด๋ค.
- โone step forwardโ๋ผ๋ ๋ง์ $p$๋ฅผ acceptํ๊ณ , ๋ค์ ์ ๋ ฌ๋ point๋ก ๋์ด๊ฐ๋ผ๋ ๋ง์ด๋ค.
// one step forward๊ฐ $r$์ accept ํ๋ ๊ฒ์ ์๋๋ค. $r$์ ์ธ์ ๋ ์ง ์ดํ์ reject ๋ ์ ์๋ค! - โremove & one step backwardโ๋ ํ์ฌ์ $\vec{pq}$์์ $q$๋ฅผ rejectํ๊ณ , ๋ง์ง๋ง์ ์ดํด๋ณธ 2๊ฐ ์ ์ผ๋ก ์ง์ ์ ๊ฐฑ์
// ๋ง์ฐฌ๊ฐ์ง๋ก ์ด๊ฒ์ $r$์ acceptํ๋ ๊ฒ์ด ์๋๋ค!
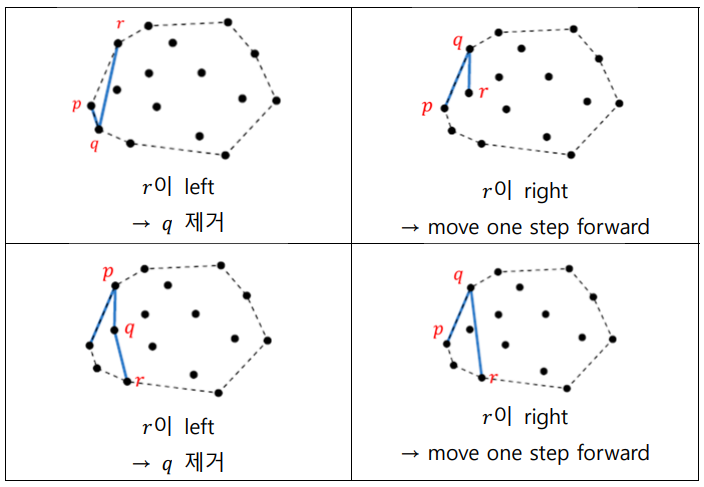
์ด ๊ณผ์ ์ ๋ฐ๋ณตํ๋ฉด, sorted list $L$์ convex hull์ ์ด๋ฃจ๋ ์ ๋ค๋ง ๋จ๊ฒ ๋๋ค!! ๐
<Grahamโs Scan>์ ์๊ฐ ๋ณต์ก๋๋ฅผ ๋ถ์ํด๋ณด์. ๊ฐ ๊ณผ์ ์ ๋ฐ๋ณต ํ์ ๋ณ๋ก ์๊ฐํด๋ณธ๋ค๋ฉด,
- rule (1)๋ง ๊ณ์ ์ ์ฉ โ ์ต๋ $n-2$๋ฒ ์ ์ฉ ๊ฐ๋ฅ!
- ๋ง์ฝ sorted list $L$์ convex hull์ด ์๋ ์ ๋ค์ด ์๋ค๋ฉด, ์ฐ๋ฆฌ๋ rule (2)๋ฅผ ํตํด ๊ทธ๋ฐ ์ ๋ค์ ์ ๊ฑฐํ๊ฒ ๋๋ค. ๋ฐ๋ผ์, rule (2)๋ ์ด $n-h$๋ฒ ์ ์ฉ๋๋ค! ($h$ = #. of upper convex hull points)
์ด๋ ๊ฒ <Grahamโs Scran>์ ํ๊ฒ ๋๋ฉด, ์ฐ๋ฆฌ๋ convex hull์ ์ ๊ป์ง์ ์ป๊ฒ ๋๋ค!! ๋๋จธ์ง ์๋ ๊ป์ง์ sorted list์ ๋ฐ๋์์๋ถํฐ rule์ ๋ค์ง์ด ์ ์ฉํ๋ฉด ์ป์ ์ ์๋ค!!
์๊ณ ๋ฆฌ์ฆ ํ ํฌ๋ ์ค์๋ <Convex hull trick>๋ผ๋ ์ต์ ํ ์๊ณ ๋ฆฌ์ฆ์ด ์๋ค. ์ฌ์ค ์ด๋ฆ์ โConvex hullโ์ด ๋ถ์์ง๋ง, <Convex hull algorithm>๊ณผ๋ ์ ํ ๋ค๋ฅธ ์ข ๋ฅ์ ์๊ณ ๋ฆฌ์ฆ์ด๋ค.
์ถ์ฒ ๋ฌธ์
- ๋ฐฑ์ค 1708๋ฒ: ๋ณผ๋ก ๊ป์ง // ์ข์ ๋ฌธ์ ๋ค. ์๋ฃ๊ตฌ์กฐ๋ค์ ๋ํ ์ดํด์ ๋ํ ์ผ์ ์ ๊ฒฝ์จ์ผ ํ๋ค.
- ๋ฐฑ์ค 4181๋ฒ: Convex Hull