Kruskalโs Algorithm & Primโs Algorithm
2020-1ํ๊ธฐ, ๋ํ์์ โ์๊ณ ๋ฆฌ์ฆโ ์์ ์ ๋ฃ๊ณ ๊ณต๋ถํ ๋ฐ๋ฅผ ์ ๋ฆฌํ ๊ธ์ ๋๋ค. ์ง์ ์ ์ธ์ ๋ ํ์์ ๋๋ค :) ์ ์ฒด ํฌ์คํธ๋ Algorithm ํฌ์คํธ์์ ํ์ธํ์ค ์ ์์ต๋๋ค.
MST๋ weighted undirected graph $G=(V, E, W)$๊ฐ ์ ๋ ฅ์ผ๋ก ๋ค์ด์ฌ ๋, ์๋์ ์์ ๋ง์กฑํ๋ tree $T=(V, Eโ)$๋ฅผ ์ถ๋ ฅํ๋ ์๊ณ ๋ฆฌ์ฆ์ด๋ค.
\[\underset{E'}{\text{argmin}} \sum_{e\in E'} w_e\]Kruskalโs Algorithm
<Kruskalโs Algorithm>์ empty graph์์ ์์ํด ์๋์ ์์๋๋ก Greedy ๋ฐฉ์์ผ๋ก edge๋ฅผ ์ ํํ๋ ์๊ณ ๋ฆฌ์ฆ์ด๋ค.
๋จ์ edge ์ค์ ๊ฐ์ฅ lightํ edge๋ฅผ ๊ทธ๋ํ์ ์ถ๊ฐํ๋ค. ๋จ, edge๋ฅผ ์ถ๊ฐํ์ ๋, ๊ทธ๋ํ์์ cycle์ด ์๊ธฐ์ง ์์์ผ ํ๋ค.
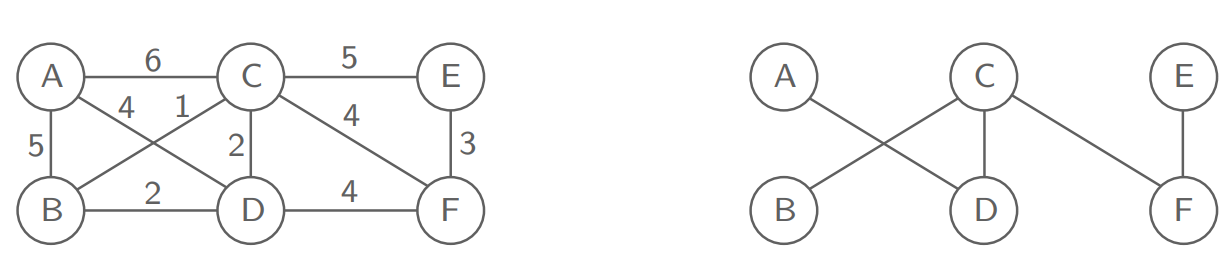
ํฌ๋ฃจ์ค์นผ ์๊ณ ๋ฆฌ์ฆ์ correctness๋ <cut property>์ ์ํด ๋ณด์ฅ๋๋ค.
Theorem. Cut Property
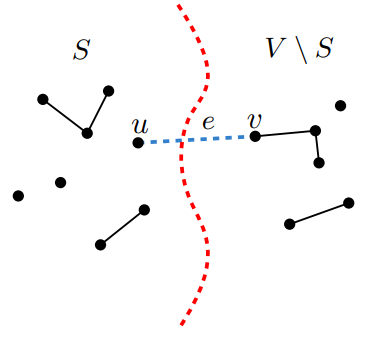
Supp. edges in $X \subset E$ are part of a MST $T$ of $G = (V, E)$.
Pick any subset of nodes $S$ for which no edge in $X$ crosses btw $S$ and $V\setminus S$,
and let $e$ be the lighstest edge across this partition.
Then $X \cup \{ e \}$ is part of some MST.
ํด์ค์ ์ข ํ์๋ฉด, $X$๊ฐ MST $T$์ ๋ถ๋ถ์งํฉ์ด๋ผ๊ณ ํ์. ์ด๋, ์ด $X$์ ๋จ ํ๋์ edge๋ฅผ ์ถ๊ฐํ์ ๋์๋ ์ฌ์ ํ MST $T$์ ๋ถ๋ถ์งํฉ์ด ๋๊ฒ ๋ง๋ค๊ณ ์ ํ๋ค. ๊ทธ๋ฌ๋ฉด, $X$์ ๋ชจ๋ edge๊ฐ cross ํ์ง ์๋๋ก ์งํฉ $S$, $V \setminus S$๋ฅผ ์ก์์ ๋, ๋ ์งํฉ์ ๊ฐ๋ก์ง๋ฅด๋ edge ์ค ๊ฐ์ฅ lightํ edge $e$๋ฅผ ์ ํํด $X$์ ์ถ๊ฐํ๋ค๋ฉด, $X \cup \{e\}$๊ฐ ์ฌ์ ํ MST์ ๋ถ๋ถ์งํฉ์ด ๋๋ค๋ ์ ๋ฆฌ์ด๋ค.
Proof.
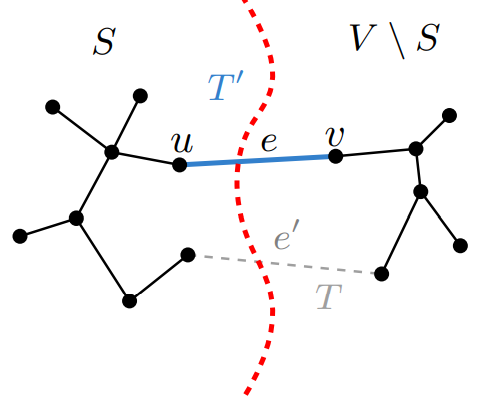
(๊ท๋ฅ๋ฒ) Assume $e = (u, v) \notin T$.
[1] ์ด๋์ $e$๋ ์์์ ์ธ๊ธํ $S$, $V\setminus S$๋ฅผ ์๋ ๊ฐ์ฅ lightํ edge๋ค.
[2] ์ด๋์ $T$๋ true MST $T$๋ฅผ ์๋ฏธํ๋ค.
We can construct a different MST $Tโ$ containing $X \cup \{ e \}$ by altering $T$ slightly.
$u$ and $v$ are connected by a path in $T$ which contains an edge $eโ$ crossing $S$ and $V\setminus S$.
Construct a new tree $Tโ$ from $T$ by removing $eโ$ and adding $e$.
Then, $Tโ$ is a spanning tree with $\texttt{cost}(Tโ) \le \texttt{cost}(T)$ (์๋ํ๋ฉด, $w_e \le w_{eโ}$์ด๊ธฐ ๋๋ฌธ.) ์ด๊ฒ์ ST $T$๊ฐ MST๋ผ๋ ์ฌ์ค์ ๋ชจ์์ด๋ค. ๋ฐ๋ผ์, ์ฒ์์ ๊ฐ์ ํ $e = (u, v) \notin T$๋ ๊ฑฐ์ง์ด๋ค.
๋ฐ๋ผ์, $S$, $V\setminus S$๋ฅผ ์ฐ๊ฒฐํ ๋, the lightest edge $e$๋ฅผ ์ถ๊ฐํ๋ ๊ฒ์ด ์ค์ ๋ก MST๊ฐ ๋จ์ด ๋ณด์ฅ๋๋ค.
<Kruskal Algorithm>์ <set> ์๋ฃํ์ ์ฌ์ฉํด ์ฝ๊ฒ ๊ตฌํํ ์ ์๋ค! ๐คฉ
Algorithm: Kruskal($G$, $w$)
($G = (V, E)$ is a connected undirected graph with edge weights $w_e$.)
// initialization
for all $u \in V$ do
โโ $\texttt{makeset}(u)$
end for
// construct empty MST
$X = \{ \}$
Sort the edges $E$ by weight.
// greedy process!
for all edges $\{ u, v\} \in E$, in increasing order of weight
โโif $\texttt{find}(u) \ne \texttt{find}(v)$ // cycle check!
โโโโadd edge $\{u, v\}$ to $X$
โโโโ$\texttt{union}(u, v)$ // merge two sets!
โโend if
end for
์๊ฐ๋ณต์ก๋๋ฅผ ์ดํด๋ณด๋ฉด,
- Weight์ ๋ฐ๋ผ ์ ๋ ฌ: $O(E \log E)$
- $\texttt{makeset}$: $V$ ๋ฒ
- $\texttt{find}$: $E$ ๋ฒ
- $\texttt{union}$: $V-1$ ๋ฒ
$\texttt{makeset}$, $\texttt{find}$, $\texttt{union}$ ์ฐ์ฐ์ ๋ํ ์๊ฐ๋ณต์ก๋๋ ์ถํ์ Disjoint Set ํฌ์คํธ์์ ์ดํด๋ณด๊ฒ ๋ค.
Primโs Algorithm
<Primโs Algorithm>์ผ๋ก๋ MST๋ฅผ ์ป์ ์ ์๋ค. ์ด ์๊ณ ๋ฆฌ์ฆ์ ์์ด๋์ด๋ ์์ฃผ ๊ฐ๋จํ๋ค!
<Primโs Algorithm>์ ๊ทธ๋ํ $G$์์ ๊ฐ์ฅ lightํ edge๋ฅผ ์ ํํ๋ฉฐ, intermediate MST $X$๋ฅผ grow ํ๋ ์๊ณ ๋ฆฌ์ฆ์ด๋ค. ์ด๋, ํฌ๋ฃจ์ค์ปฌ ๋์ ๋ง์ฐฌ๊ฐ์ง๋ก ์ ํํ edge๋ก ์ธํด cycle์ด ํ์ฑ๋์ด์๋ ์ ๋๋ค.
Algorithm: Prime($G$, $w$)
($G = (V, E)$ is a connected undirected graph with edge weights $w_e$.)
// initialization
for all $u \in V$
โโ$\texttt{cost}(u) = \infty$, and $\texttt{prev}(u)=\texttt{nil}$
Pick any initial node $u_0$ and set $\texttt{cost}(u_0) = 0$ // ๋น์ฐํ๊ฒ๋ ์ด๋ค ๋ ธ๋๋ฅผ ์์์ผ๋ก ์ผ๋ ์ ํ ์๊ด์ด ์๋ค!
$H=\texttt{makequeue}(V)$ // priority queue, using (cost, value) pair
// greedy process!
while $H$ is not empty
โโ$v=\texttt{deletemin}(H)$
โโfor each $\{v, z\} \in E$
โโโโif $\texttt{cost}(z) > w(v, z)$
โโโโโโ$\texttt{cost}(z) = w(v, z)$, and $\texttt{prev}(z) = v$
โโโโโโ$\texttt{decreasekey}(H, z)$
ํฌ๋ฃจ์ค์ปฌ ์๊ณ ๋ฆฌ์ฆ๊ณผ ๋ฌ๋ฆฌ ์ ๋ ฌ์ด ์๊ธฐ ๋๋ฌธ์, ํ๋ฆผ ์๊ณ ๋ฆฌ์ฆ์ ์๊ฐ๋ณต์ก๋๋ Priority Queue๋ฅผ ์ฐ๋๋ฐ ๊ฑธ๋ฆฌ๋ $E \times O(\log V) = O(E \log V)$์ด๋ค.
ํ๋ฆผ ์๊ณ ๋ฆฌ์ฆ์ ํํ๋ฅผ ์ ์ดํด๋ณด๋ฉด, ์์์ ๋ดค๋ Dijkstraโs Algorithm๊ณผ ์๋นํ ๋น์ทํ๋ค! ๋์ ์ฐจ์ด์ ์ $\texttt{cost}(\cdot)$ ์ ๋ฐ์ดํธ rule์์ ์๋๋ฐ,
๋ค์ต์คํธ๋ผ ์๊ณ ๋ฆฌ์ฆ์์ ์ถ๋ฐ ๋ ธ๋ $s$์์ ๋๋ฌํ๋๋ฐ ๋๋ ์ต์ ๋น์ฉ์ $\texttt{cost}(\cdot)$์ ๊ธฐ๋กํ๊ณ , ํ๋ฆผ ์๊ณ ๋ฆฌ์ฆ์์๋ ๋ ธ๋ $v$๋ฅผ MST์ ํฌํจ์ํฌ ๋์ ๋น์ฉ์ $\texttt{cost}(\cdot)$์ ๊ธฐ๋กํ๋ค.
ํฌ๋ฃจ์ค์ปฌ๊ณผ ํ๋ฆผ์ ๋น๊ตํ๋ค๋ฉด, ๊ฐ์ธ์ ์ผ๋ก ํ๋ฆผ์ด ๋ ์ฌ์ด ์๊ณ ๋ฆฌ์ฆ์ด๋ผ๊ณ ์๊ฐํ๋ค. ์๋ํ๋ฉด, ํ๋ฆผ์์๋ cycle์ ํ๋จํ ํ์๋ ์๊ณ , ์ ๋ง greedy์ ์ถฉ์คํ๊ฒ ๋ ธ๋๋ฅผ ์ ํํด๋๊ฐ๋ฉด ๋๊ธฐ ๋๋ฌธ์ด๋ค!
์ด์ด์ง๋ ํฌ์คํธ์์๋ <Kruskalโs Algorithm>์์ ์ธ๊ธ๋์๋ <Disjoint Set>์ ๋ํด ์ดํด๋ณธ๋ค. ์ด ๋ถ๋ถ์ Greedy Algorithm๊ณผ ์ง์ ์ ์ผ๋ก ์ฐ๊ด๋ ๋ถ๋ถ์ ์๋๋ฉฐ, <Disjoint Set>์ด๋ผ๋ ์๋ฃ๊ตฌ์กฐ๋ฅผ ์ด๋ป๊ฒ ๊ตฌํํ ์ ์๊ณ , ๊ทธ๋ฆฌ๊ณ ๊ทธ๋์ ์ฌ์ฉ๋๋ ํ ํฌ๋์ ๋ํด ๋ค๋ฃฌ๋ค.
๐ Disjoint Set